C Program to Check Whether a Number is Prime or Not
Example to check whether an integer (entered by the user) is a prime number or not using for loop and if...else statement.
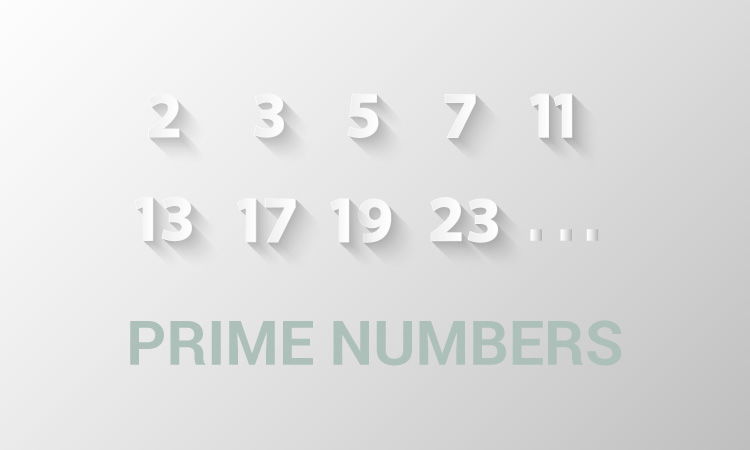
To understand this example, you should have the knowledge of following C programming topics:
A prime number is a positive integer which is divisible only by 1 and itself. For example: 2, 3, 5, 7, 11, 13
Example: Program to Check Prime Number
#include
int main()
{
int n, i, flag = 0;
printf("Enter a positive integer: ");
scanf("%d", &n);
for(i = 2; i <= n/2; ++i)
{
// condition for nonprime number
if(n%i == 0)
{
flag = 1;
break;
}
}
if (n == 1)
{
printf("1 is neither a prime nor a composite number.");
}
else
{
if (flag == 0)
printf("%d is a prime number.", n);
else
printf("%d is not a prime number.", n);
}
return 0;
}
Output
Enter a positive integer: 29 29 is a prime number.
If the
for
loop terminates when the test expression of loop i <= n/2
is false, the entered number is a prime number. The value of flag is equal to 0 in this case.
If the loop terminates because of
break
statement inside the if
statement, the entered number is a nonprime number. The value of flag is 1 in this case.C Program to Check Whether a Number can be Expressed as Sum of Two Prime Numbers
Example to check if an integer (entered by the user) can be expressed as the sum of two prime numbers of all possible combinations with the use of functions.
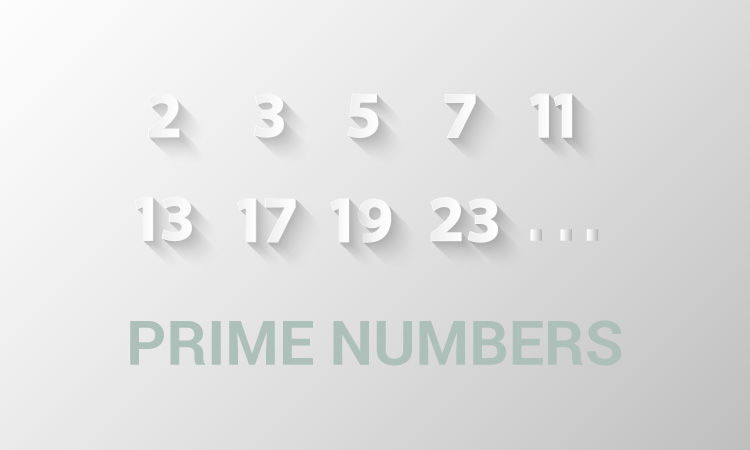
To understand this example, you should have the knowledge of following C programming topics:
To accomplish this task,
checkPrime()
function is created.
The
checkPrime()
returns 1 if the number passed to the function is a prime number. Example: Integer as a Sum of Two Prime Numbers
#include
int checkPrime(int n);
int main()
{
int n, i, flag = 0;
printf("Enter a positive integer: ");
scanf("%d", &n);
for(i = 2; i <= n/2; ++i)
{
// condition for i to be a prime number
if (checkPrime(i) == 1)
{
// condition for n-i to be a prime number
if (checkPrime(n-i) == 1)
{
// n = primeNumber1 + primeNumber2
printf("%d = %d + %d\n", n, i, n - i);
flag = 1;
}
}
}
if (flag == 0)
printf("%d cannot be expressed as the sum of two prime numbers.", n);
return 0;
}
// Function to check prime number
int checkPrime(int n)
{
int i, isPrime = 1;
for(i = 2; i <= n/2; ++i)
{
if(n % i == 0)
{
isPrime = 0;
break;
}
}
return isPrime;
}
Output
Enter a positive integer: 34 34 = 3 + 31 34 = 5 + 29 34 = 11 + 23
34 = 17 + 17
C Program to Find the Largest Number Among Three Numbers
In this example, the largest number among three numbers (entered by the user) is found using three different methods.To understand this example, you should have the knowledge of following C programming topics:This program uses only if statement to find the largest number.Example #1
#include
int main()
{
double n1, n2, n3;
printf("Enter three different numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
if( n1>=n2 && n1>=n3 )
printf("%.2f is the largest number.", n1);
if( n2>=n1 && n2>=n3 )
printf("%.2f is the largest number.", n2);
if( n3>=n1 && n3>=n2 )
printf("%.2f is the largest number.", n3);
return 0;
}
This program uses if...else statement to find the largest number.Example #2
#include
int main()
{
double n1, n2, n3;
printf("Enter three numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
if (n1>=n2)
{
if(n1>=n3)
printf("%.2lf is the largest number.", n1);
else
printf("%.2lf is the largest number.", n3);
}
else
{
if(n2>=n3)
printf("%.2lf is the largest number.", n2);
else
printf("%.2lf is the largest number.",n3);
}
return 0;
}
This program uses nested if...else statement to find the largest number.Example #3
#include
int main()
{
double n1, n2, n3;
printf("Enter three numbers: ");
scanf("%lf %lf %lf", &n1, &n2, &n3);
if( n1>=n2 && n1>=n3)
printf("%.2lf is the largest number.", n1);
else if (n2>=n1 && n2>=n3)
printf("%.2lf is the largest number.", n2);
else
printf("%.2lf is the largest number.", n3);
return 0;
}
Though, the largest number among three numbers is found using multiple ways, the output of all these program will be same.Enter three numbers: -4.5 3.9 5.6 5.60 is the largest number.C Program to Find LCM of two Numbers
Examples on different ways to calculate the LCM (Lowest Common Multiple) of two integers using loops and decision making statements.To understand this example, you should have the knowledge of following C programming topics:The LCM of two integers n1 and n2is the smallest positive integer that is perfectly divisible by both n1 and n2(without a remainder). For example: the LCM of 72 and 120 is 360.Example #1: LCM using while Loop and if Statement
#include
int main()
{
int n1, n2, minMultiple;
printf("Enter two positive integers: ");
scanf("%d %d", &n1, &n2);
// maximum number between n1 and n2 is stored in minMultiple
minMultiple = (n1>n2) ? n1 : n2;
// Always true
while(1)
{
if( minMultiple%n1==0 && minMultiple%n2==0 )
{
printf("The LCM of %d and %d is %d.", n1, n2,minMultiple);
break;
}
++minMultiple;
}
return 0;
}
OutputEnter two positive integers: 72 120 The LCM of 72 and 120 is 360.In this program, the integers entered by the user are stored in variable n1and n2 respectively.The largest number among n1 and n2 is stored in minMultiple. The LCM of two numbers cannot be less than minMultiple.The test expression of while loop is always true (1). In each iteration, whether minMultiple is perfectly divisible by n1 and n2 is checked. If this test condition is not true, minMultiple is incremented by 1 and the iteration continues until the test expression of if statement is true.The LCM of two numbers can also be found using the formula:LCM = (num1*num2)/GCDLearn more on, how to find the GCD of two numbers in C programming.Example #2: LCM Calculation by Finding GCD
#include
int main()
{
int n1, n2, i, gcd, lcm;
printf("Enter two positive integers: ");
scanf("%d %d",&n1,&n2);
for(i=1; i <= n1 && i <= n2; ++i)
{
// Checks if i is factor of both integers
if(n1%i==0 && n2%i==0)
gcd = i;
}
lcm = (n1*n2)/gcd;
printf("The LCM of two numbers %d and %d is %d.", n1, n2, lcm);
return 0;
}
C Program to Calculate the Sum of Natural Numbers
To compute the sum of natural numbers from 1 to n (entered by the user), loops can be used. You will learn how to use for loop and while loop to solve this problem.To understand this example, you should have the knowledge of following C programming topics:The positive numbers 1, 2, 3... are known as natural numbers. The programs below takes a positive integer (let say n) as an input from the user and calculates the sum up to n.Example #1: Sum of Natural Numbers Using for Loop
#include
int main()
{
int n, i, sum = 0;
printf("Enter a positive integer: ");
scanf("%d",&n);
for(i=1; i <= n; ++i)
{
sum += i; // sum = sum+i;
}
printf("Sum = %d",sum);
return 0;
}
The above program takes the input from the user and stores in variable n. Then, for loop is used to calculate the sum upto the given number.Example #2: Sum of Natural Numbers Using while Loop
#include
int main()
{
int n, i, sum = 0;
printf("Enter a positive integer: ");
scanf("%d",&n);
i = 1;
while ( i <=n )
{
sum += i;
++i;
}
printf("Sum = %d",sum);
return 0;
}
OutputEnter a positive integer: 100 Sum = 5050In both programs, the loop is iterated n number of times. And, in each iteration, the value of i is added to sum and i is incremented by 1.Though both programs are technically correct, it is better to use for loop in this case. It's because the number of iteration is known.The above programs doesn't work properly if the user enters a negative integer. Here's a little modification of the above program to take input from the user until positive integer is entered.Example #3: Program to Read Input Until User Enters a Positive Integer
#include
int main()
{
int n, i, sum = 0;
do {
printf("Enter a positive integer: ");
scanf("%d",&n);
}
while (n <= 0);
for(i=1; i <= n; ++i)
{
sum += i; // sum = sum+i;
}
printf("Sum = %d",sum);
return 0;
Very good experience
ReplyDelete💞💞💞
ReplyDelete₹=100k
ReplyDeletebetter
ReplyDeletekaha ho Guru badiya
ReplyDelete